Image Frame A
Image with background color.
Preview
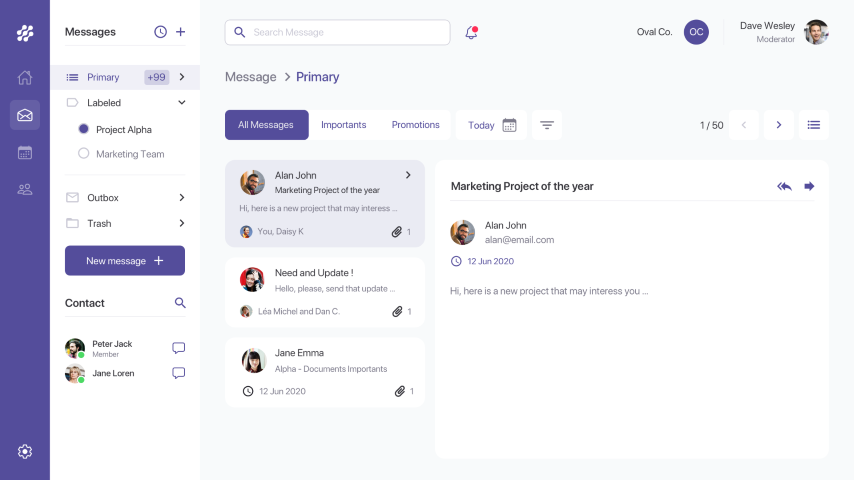
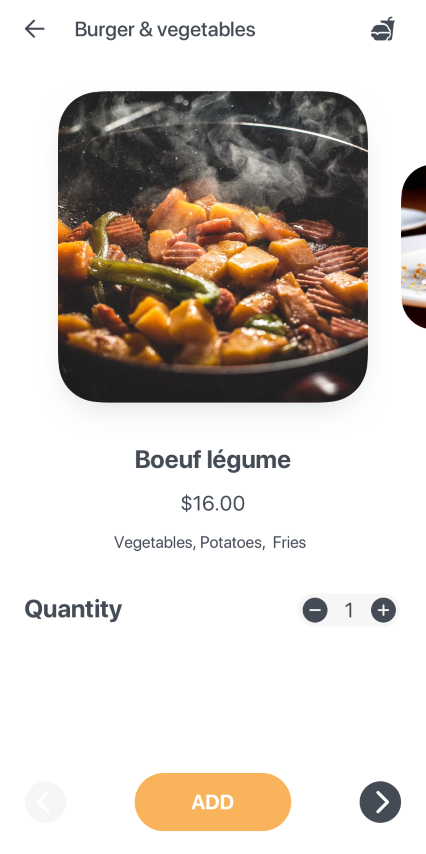
Code
import React from "react"; import styles from "./image-frame-a.module.css"; type Props = { className?: string; title?: React.ReactNode; alt: string; src?: string; aspect?: "default" | "square" | "portrait" | "landscape"; imageAspect?: "square" | "portrait" | "landscape"; shadow?: "none" | "medium"; rounded?: "none" | "small" | "medium" | "large"; imageRounded?: "none" | "small" | "medium" | "large"; // Put additional variants here, then define them in the CSS variant?: "default" | "red"; }; export default function ImageFrameA({ className, title, alt, src, aspect = "square", imageAspect = "square", shadow = "medium", rounded = "medium", imageRounded = "medium", variant = "default", }: Props) { return ( <div className={[ styles["wrapper"], styles[`ar-${aspect}`], styles[`r-${rounded}`], styles[`sh-${shadow}`], styles[`variant-${variant}`], className, ].join(" ")} > <figure className={styles["image-wrapper"]}> {/* If you use Next.js, replace 'img' with 'Image' element */} <img className={[styles["image"], styles[`img-r-${imageRounded}`], styles[`img-ar-${imageAspect}`]].join(" ")} src={src} alt={alt} width={360} height={360} /> {title && <legend className={styles["legend"]}>{title}</legend>} </figure> </div> ); }
Design
Figma design file:
Documentation
Properties
Props of the component:
className
(string): Specifies the CSS class of the component.title
(string or ReactNode): Specifies the text that will be used as the title.alt
(string): Specifies the text that will be used as the alt attribute of the image.src
(string): Specifies the URL of the image.aspect
("default" | "square" | "portrait" | "landscape"): Specifies the aspect ratio of the frame.imageAspect
("square" | "portrait" | "landscape"): Specifies the aspect ratio of the image.shadow
("small" | "medium"): Specifies the size of the shadow of the frame.rounded
("none" | "small" | "medium" | "large"): Specifies the border radius of the frame.imageRounded
("none" | "small" | "medium" | "large"): Specifies the border radius of the image.variant
("default" | "red" | "card" or a customized value): Specifies the color or theme variant of the component. Check out the "Sample CSS customization" below for an example of how to use it.
Sample CSS customization
.variant-red { border: none; background-image: linear-gradient(to top right, #8d2164, #f83333); }