Image Frame B
Three pictures layout.
Preview
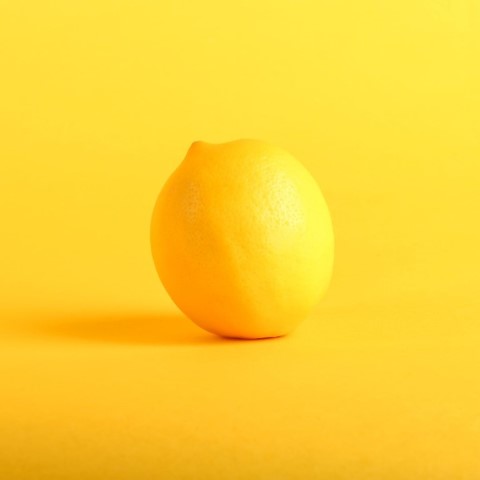
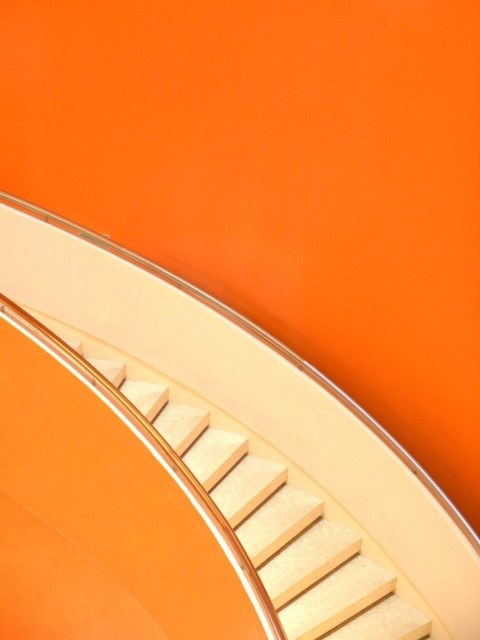
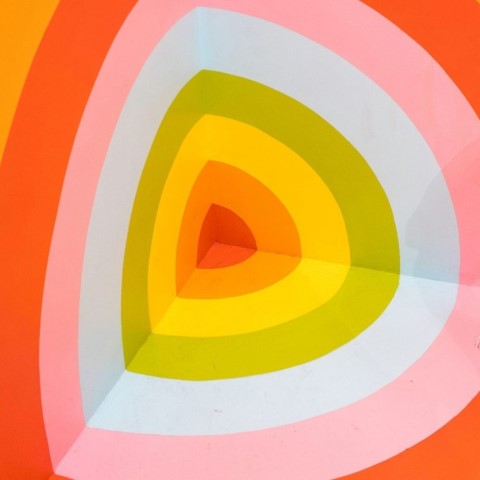
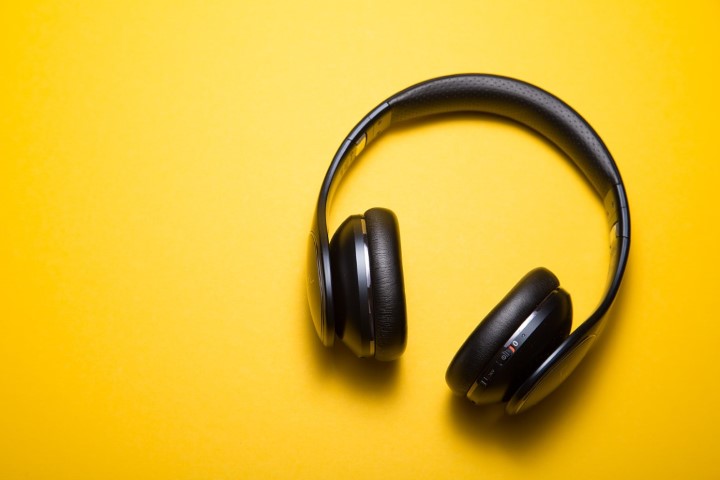
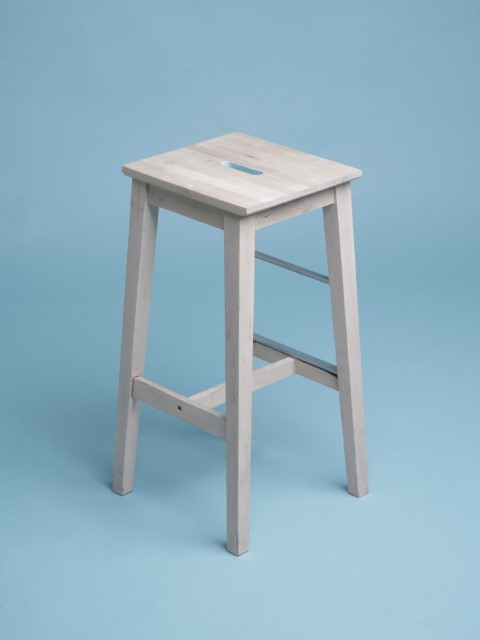
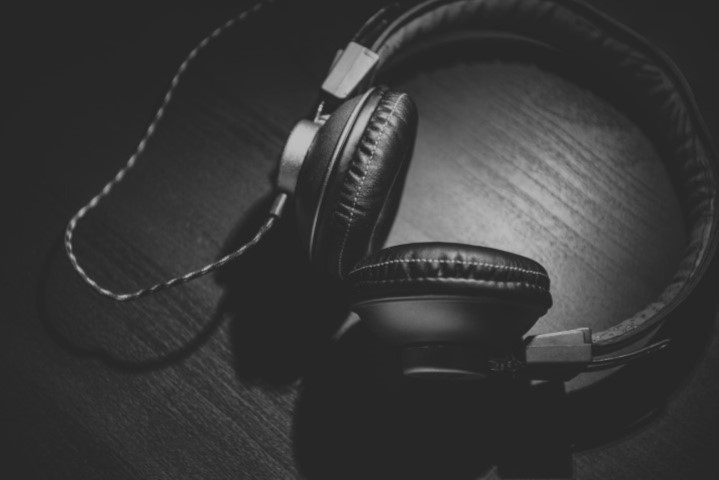
Code
import React from "react"; import styles from "./image-frame-b.module.css"; type Props = { className?: string; alt: string; imgMainSrc: string; imgTopSrc?: string; imgBottomSrc?: string; imgTopAspect?: "default" | "square" | "portrait" | "landscape"; imgBottomAspect?: "default" | "square" | "portrait" | "landscape"; rounded?: "none" | "small" | "medium" | "large"; }; export default function ImageFrameB({ className, alt, imgMainSrc, imgTopSrc, imgBottomSrc, imgTopAspect = "square", imgBottomAspect = "square", rounded = "small", }: Props) { return ( <div className={[styles["wrapper"], className].join(" ")}> <figure className={styles["image-wrapper"]}> {/* If you use Next.js, replace 'img' with 'Image' element */} <img className={[ styles["image-top"], styles[`r-${rounded}`], styles[`img-top-ar-${imgTopAspect}`], ].join(" ")} src={imgTopSrc} alt={alt} width={360} height={360} /> <img className={[styles["image"], styles[`r-${rounded}`]].join(" ")} src={imgMainSrc} alt={alt} width={360} height={360} /> <img className={[ styles["image-bottom"], styles[`r-${rounded}`], styles[`img-bottom-ar-${imgBottomAspect}`], ].join(" ")} src={imgBottomSrc} alt={alt} width={360} height={360} /> </figure> </div> ); }
Design
Figma design file:
Documentation
Properties
Props of the component:
className
(string): Specifies the CSS class of the component.alt
(string): Specifies the text that will be used as the alt attribute of the image.imgMainSrc
(string): Specifies the URL of the main image.imgTopSrc
(string): Specifies the URL of the image at top.imgBottomSrc
(string): Specifies the URL of the image at bottom.imgTopAspect
("default" | "square" | "portrait" | "landscape"): Specifies the aspect ratio of the image at top.imgBottomAspect
("default" | "square" | "portrait" | "landscape"): Specifies the aspect ratio of the image at bottom.rounded
("none" | "small" | "medium" | "large"): Specifies the border radius of the images.